Markdown Overview Buttons Tabs Footnotes Collapse ToC Navigation Formula Page-Specific Meta Custom Components
Lets create a simple Card
component
and add it to our markdowns. Let's first create the two following files:
1link$.codedoc/components/card/index.tsx # --> main component code
2link$.codedoc/components/card/style.ts # --> component styles
Codedoc uses CONNECTIVE SDH for its component system, alongside CONNECTIVE JSS themes for theming (which is in turn based on JSS). As a result, a simple cards document would look like this:
1linkimport { ThemedComponentThis } from '@connectv/jss-theme'; // @see [CONNECTIVE JSS Theme](https://github.com/CONNECT-platform/connective-jss-theme)
2linkimport { RendererLike } from '@connectv/html'; // @see [CONNECTIVE HTML](https://github.com/CONNECT-platform/connective-html)
3linkimport { CodedocTheme } from '@codedoc/core'; // --> Type helper for theme object
4link
5linkimport { CardStyle } from './style'; // @see tab:style.ts
6link
7link
8linkexport interface CardOptions { // --> a nice interface for possible props
9link raise: string; // --> which is the raise level of our cards. Note that all props MUST be of type `string`
10link}
11link
12link
13linkexport function Card(
14link this: ThemedComponentThis, // --> keep typescript strict typing happy
15link options: CardOptions, // --> the component props (attributes)
16link renderer: RendererLike<any, any>, // --> our beloved renderer
17link content: any, // --> the content of the component
18link) {
19link const classes = this.theme.classes(CardStyle); // --> fetch the theme-based classes
20link let raise = 'raised-0';
21link if (options && options.raise === '1') raise = 'raised-1'; // --> determine the proper raise level based on given attributes
22link if (options && options.raise === '2') raise = 'raised-2';
23link
24link return <div class={`${classes.card} ${raise}`}>
25link {content}
26link </div>;
27link}
1linkimport { themedStyle } from '@connectv/jss-theme'; // @see [Connective JSS Theme](https://github.com/CONNECT-platform/connective-jss-theme)
2linkimport { CodedocTheme } from '@codedoc/core';
3link
4link
5linkexport const CardStyle = themedStyle<CodedocTheme>(theme => ({
6linkcard: {
7link display: 'inline-block',
8link verticalAlign: 'middle',
9link borderRadius: 8,
10link padding: 8,
11link maxWidth: 320,
12link margin: 16,
13link overflow: 'hidden',
14link cursor: 'pointer',
15link transition: 'box-shadow .3s, transform .3s',
16link
17link '&.raised-0': { boxShadow: '0 1px 3px rgba(0, 0, 0, .12)' }, // --> different styles for different raise-levels
18link '&.raised-1': { boxShadow: '0 3px 6px rgba(0, 0, 0, .18)' },
19link '&.raised-2': { boxShadow: '0 6px 18px rgba(0, 0, 0, .25)' },
20link '&:hover': {
21link boxShadow: '0 6px 18px rgba(0, 0, 0, .25)',
22link transform: 'translateY(-8px)'
23link },
24link
25link '& img': {
26link margin: -8,
27link marginTop: -24,
28link width: 'calc(100% + 16px)',
29link maxWidth: 'none',
30link },
31link
32link '& strong': {
33link fontSize: 18,
34link display: 'block',
35link color: theme.light.primary, // --> so lets make the title's of the primary color
36link 'body.dark &': { color: theme.dark.primary }, // --> but also do respect dark-mode settings
37link '@media (prefers-color-scheme: dark)': { // --> this is to ensure proper dark-mode colors even before the scripts are loaded and user overrides are fetched
38link 'body:not(.dark-mode-animate) &': {
39link color: theme.dark.primary,
40link },
41link },
42link },
43link }
44link}));
To add this card component to our codedoc config, lets modify .codedoc/config.ts
like this:
1link
2linkimport {
3link configuration,
4link DefaultMarkdownCustomComponents // --> make sure to import the default components
5link} from '@codedoc/core';
6link
7linkimport { theme } from './theme';
8linkimport { Card } from './components/card'; // --> import the card component itself
9link
10link
11linkexport const config = /*#__PURE__*/configuration({
12link // ...
13link markdown: { // --> update markdown config
14link customComponents: { // --> add to custom components
15link ...DefaultMarkdownCustomComponents, // --> make sure to add default markdown components. otherwise the default components will not work!
16link Card, // --> add our own card component
17link }
18link },
19link // ...
20link});
Now we can use our card component in our markdown files:
1link> :Card
2link>
3link> 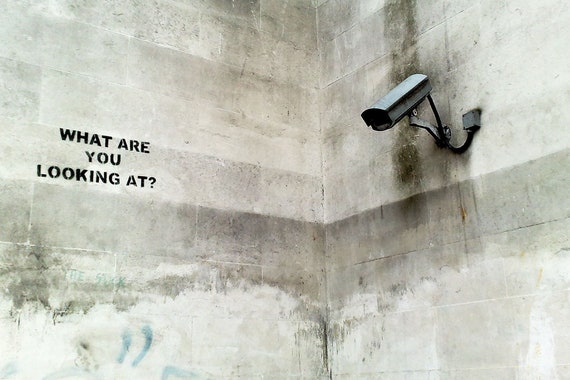
4link>
5link> **Banksy**
6link> Banksy is an anonymous England-based street artist, vandal, political activist, and film director, active since the 1990s.
7link> His satirical street art and subversive epigrams combine dark humour with graffiti executed in a distinctive stenciling technique.
8link> > :Buttons
9link> > > :Button label=Wiki, url=https://en.wikipedia.org/wiki/Banksy
10link
11link> :Card raise=1
12link>
13link> 
14link>
15link> **Jackson Pollock**
16link> Paul Jackson Pollock was an American painter and a major figure in the abstract expressionist movement.
17link> He was widely noticed for his technique of pouring or splashing liquid household paint onto a horizontal surface,
18link> enabling him to view and paint his canvases from all angles
19link> > :Buttons
20link> > > :Button label=Wiki, url=https://en.wikipedia.org/wiki/Jackson_Pollock
Banksy Banksy is an anonymous England-based street artist, vandal, political activist, and film director, active since the 1990s. His satirical street art and subversive epigrams combine dark humour with graffiti executed in a distinctive stenciling technique.
Jackson Pollock Paul Jackson Pollock was an American painter and a major figure in the abstract expressionist movement. He was widely noticed for his technique of pouring or splashing liquid household paint onto a horizontal surface, enabling him to view and paint his canvases from all angles
You can similarly create your own inline custom components:
1linkimport { ThemedComponentThis } from '@connectv/jss-theme';
2linkimport { RendererLike } from '@connectv/html';
3linkimport { CodedocTheme } from '@codedoc/core';
4link
5linkimport { TagStyle } from './style'; // @see tab:Style Code
6link
7link
8linkexport function Tag(
9link this: ThemedComponentThis<CodedocTheme>,
10link _: any,
11link renderer: RendererLike<any, any>,
12link content: any
13link) {
14link const classes = this.theme.classes(TagStyle);
15link return <span class={classes.tag}># {content}</span>
16link}
1linkimport { themedStyle } from '@connectv/jss-theme';
2linkimport { CodedocTheme } from '@codedoc/core';
3link
4link
5linkexport const TagStyle = themedStyle<CodedocTheme>(theme => ({
6link tag: {
7link display: 'inline-flex',
8link alignItems: 'center',
9link verticalAlign: 'middle',
10link height: 16,
11link borderRadius: 16,
12link padding: 8,
13link background: theme.light.primary,
14link color: theme.light.primaryContrast,
15link
16link 'body.dark &': {
17link background: theme.dark.primary,
18link color: theme.dark.primaryContrast,
19link },
20link }
21link}));
1linkimport {
2link configuration,
3link DefaultMarkdownCustomInlineComponents // --> make sure to import the default components
4link} from '@codedoc/core';
5link
6linkimport { theme } from './theme';
7linkimport { Tag } from './components/tag'; // --> import the tag component itself
8link
9link
10linkexport const config = /*#__PURE__*/configuration({
11link // ...
12link markdown: { // --> update markdown config
13link customInlineComponents: { // --> add to custom components
14link ...DefaultMarkdownInlineCustomComponents, // --> make sure to add default markdown components. otherwise the default components will not work!
15link Tag, // --> add our own tag component
16link }
17link },
18link // ...
19link});
Which can be used like this:
1link<!-- ... -->
2linkAnd now lets use [Some Tags](:Tag) inside our text.
3link<!-- ... -->
And now lets use # Some Tags inside our text.